Understanding JavaScript Symbols: Beyond Strings and Numbers
JavaScript Symbols are unique, immutable identifiers used as object keys. They help avoid naming collisions, enable hidden properties, and allow powerful features like custom iteration. They’re crucial in frameworks like React or Vue, powering safer, more robust JavaScript code.
What Are Symbols and Why They Matter
Symbols are a special data type introduced in JavaScript with ECMAScript 2015 (ES6). Unlike string
, number
, or boolean
, Symbols
create completely unique identifiers. They’re particularly useful for avoiding naming conflicts and enabling advanced customization of objects.
Think of Symbols as uncopyable keys — each created with its own unique fingerprint. They’re a key addition to the language for writing safer, more expressive, and collision-free code.
Key Characteristics
- Uniqueness:
Each Symbol is guaranteed to be unique, even if multiple Symbols share the same description. - Immutability:
Once created, a Symbol can’t be changed, ensuring its reliability. - Non-enumerable:
Symbol-keyed properties don’t show up in normal object enumeration methods likefor...in
orObject.keys()
.
Why They Matter
- Avoid Property Name Collisions:
Their uniqueness solves naming conflicts in large codebases. - Enable Advanced Language Features:
They allow customization of iteration, type conversion, and other behaviors via well-known Symbols. - Power Framework Internals:
Symbols are widely used in frameworks like React, Redux, and Vue to store private metadata, reducing the risk of collisions.
A Brief History of Symbols
Before ES6, JavaScript objects only used strings as property keys. This increased the risk of accidental collisions. Developers resorted to conventions like _privateVar
or closures to simulate private properties.
Symbols were introduced to:
- Provide safe extension of objects without collision.
- Enable customization of language behaviors (like iteration, type coercion, etc.) via well-known symbols.
Shortly after their introduction, major frameworks like React began using Symbols to attach internal data without conflicting with user-defined properties.
Symbol Basics
Creating a Symbol
You can create a Symbol using the Symbol()
function. Each call generates a unique Symbol:
const mySymbol = Symbol();
console.log(typeof mySymbol); // "symbol"
Optionally, you can add a description for easier debugging:
const colorSymbol = Symbol('color');
console.log(colorSymbol.description); // "color"
Pro Tip: Always use descriptive names for easier debugging in complex applications.
Using Symbols as Object Keys
Symbols can be used as unique property keys:
const sizeSymbol = Symbol('size');
const product = {
[sizeSymbol]: 'Large'
};
console.log(product[sizeSymbol]); // "Large"
console.log(Object.keys(product)); // [] - Symbol keys don’t appear in standard enumeration
To explicitly list Symbols, use Object.getOwnPropertySymbols()
:
console.log(Object.getOwnPropertySymbols(product)); // [ Symbol(size) ]
Use Cases and Examples
Unique Property Keys
Symbols help avoid property name collisions in large codebases:
const TYPE = Symbol('type');
class Widget {
constructor(name) {
this.name = name;
this[TYPE] = 'Widget';
}
}
const widget = new Widget('Menu');
widget.type = 'CustomString';
console.log(widget.type); // "CustomString" - User-defined property
console.log(widget[TYPE]); // "Widget" - Symbol property avoids conflict
Emulating Private Properties
While modern JavaScript now has private class fields (#property
), Symbols are still used to hide implementation details:
const _secret = Symbol('secret');
class User {
constructor(name, password) {
this.name = name;
this[_secret] = password;
}
checkPassword(password) {
return this[_secret] === password;
}
}
const user = new User('Alice', 'mypassword');
console.log(user.checkPassword('mypassword')); // true
Creating Enums with Symbols
Symbols make robust, collision-free enums:
const STATUS = {
PENDING: Symbol('pending'),
IN_PROGRESS: Symbol('in_progress'),
COMPLETED: Symbol('completed'),
};
function getStatusMessage(status) {
switch (status) {
case STATUS.PENDING:
return 'Task is pending...';
case STATUS.IN_PROGRESS:
return 'Task is in progress.';
case STATUS.COMPLETED:
return 'Task is completed!';
default:
return 'Unknown status.';
}
}
console.log(getStatusMessage(STATUS.PENDING)); // "Task is pending..."
Using Well-Known Symbols
ES6 introduced “well-known symbols” that let you customize object behavior in language-level operations.
Common ones include Symbol.iterator
, Symbol.toStringTag
, and Symbol.hasInstance
.
Example: Making an Object Iterable
const range = {
start: 1,
end: 5,
[Symbol.iterator]() {
let current = this.start;
const end = this.end;
return {
next() {
if (current <= end) {
return { value: current++, done: false };
}
return { done: true }; // End of iteration
},
};
},
};
for (const num of range) {
console.log(num); // Logs: 1, 2, 3, 4, 5
}
You can also customize toString()
with Symbol.toStringTag
:
class CustomClass {
get [Symbol.toStringTag]() {
return 'CustomClass';
}
}
const instance = new CustomClass();
console.log(Object.prototype.toString.call(instance));
// "[object CustomClass]"
Symbol Registry (Symbol.for
and Symbol.keyFor
)
A global symbol registry lets you create/retrieve the same symbol by name:
const globalSymbolA = Symbol.for('myGlobal');
const globalSymbolB = Symbol.for('myGlobal');
console.log(globalSymbolA === globalSymbolB); // true
console.log(Symbol.keyFor(globalSymbolA)); // "myGlobal"
This is helpful when you want to share symbols across different parts of an application or across iframes.
How Frameworks Use Symbols (Examples)
Many popular JavaScript frameworks leverage symbols internally for metadata, avoiding collisions with user properties.
React
Under the hood, React uses global symbols to identify the type of an element (Symbol.for('react.element')
, Symbol.for('react.portal')
, etc.).
Here’s a simplified example showing how React can check if something is a valid React element by comparing its internal $$typeof
property to a shared symbol:
// React internally uses something like this:
const REACT_ELEMENT_TYPE = Symbol.for('react.element');
function isReactElement(obj) {
return (
typeof obj === 'object' &&
obj !== null &&
obj.$$typeof === REACT_ELEMENT_TYPE
);
}
// Example usage:
const myObj = {
$$typeof: Symbol.for('react.element'),
type: 'div',
props: { children: 'Hello World' },
};
console.log(isReactElement(myObj));
// true, because $$typeof matches Symbol.for('react.element')
Using a globally registered symbol ensures any environment or bundle recognizes React elements consistently.
Redux
In Redux, you can find or build custom middleware that uses symbols for internal metadata, ensuring they don’t conflict with string-based action types:
// Custom middleware that checks for an "internal" symbol on an action.
const INTERNAL_ACTION = Symbol('INTERNAL_ACTION');
function internalActionMiddleware(store) {
return function (next) {
return function (action) {
if (action[INTERNAL_ACTION]) {
// process internal action
console.log('Received an internal action:', action.type);
// Possibly handle it differently or store internal data
}
return next(action);
};
};
}
// Usage in a Redux-like setup:
const internalAction = {
type: 'TEST_ACTION',
[INTERNAL_ACTION]: true, // Symbol-keyed
};
store.dispatch(internalAction);
// Logs: "Received an internal action: TEST_ACTION"
By using a symbol key (INTERNAL_ACTION
), we ensure there’s no clash with user-defined strings for type
.
Vue
In Vue 3, symbols are often used to define unique injection keys for the provide/inject
feature:
// Symbol as a key for dependency injection in Vue 3
// Provide (in a parent component)
import { provide } from 'vue';
const myInjectionKey = Symbol('myInjectionKey');
export default {
setup() {
provide(myInjectionKey, 'Hello from parent!');
}
};
// Inject (in a child component)
import { inject } from 'vue';
export default {
setup() {
const message = inject(myInjectionKey);
console.log(message); // "Hello from parent!"
}
};
Using symbols for injection keys prevents naming collisions across different parts of an application or third-party libraries.
Best Practices for Using Symbols
- Use Descriptions
Always provide a description to assist with debugging. - Local Symbols vs. Global Registry
- Use
Symbol()
for one-off unique property keys in modules or classes. - Use
Symbol.for()
when you explicitly want a shared, reusable symbol across different parts of your app.
- Use
- Don’t Overuse
Too many Symbol properties can complicate debugging..Use them when uniqueness or “pseudo-private” logic truly matters. - Leverage Well-Known Symbols
Explore how you can customize iteration, string conversion, and other behaviors with built-in Symbols.
Conclusion and Next Steps
Symbols add:
- Collision-Free Keys
A better way to handle property name conflicts. - Enhanced Language Features
Unlock advanced object behaviors like iteration and string conversion. - Framework Internals
Store private metadata invisibly and safely.
Where to Go from Here
- Experiment with Well-Known Symbols like
Symbol.hasInstance
,Symbol.toPrimitive
, andSymbol.species
. - Dive Deeper into Framework Source Code to see real-world Symbol usage, especially in React and Redux.
- Try Symbol-based Data Structures (e.g., custom iterators, enumerations) to learn how they can improve application safety and clarity.
Further Readings
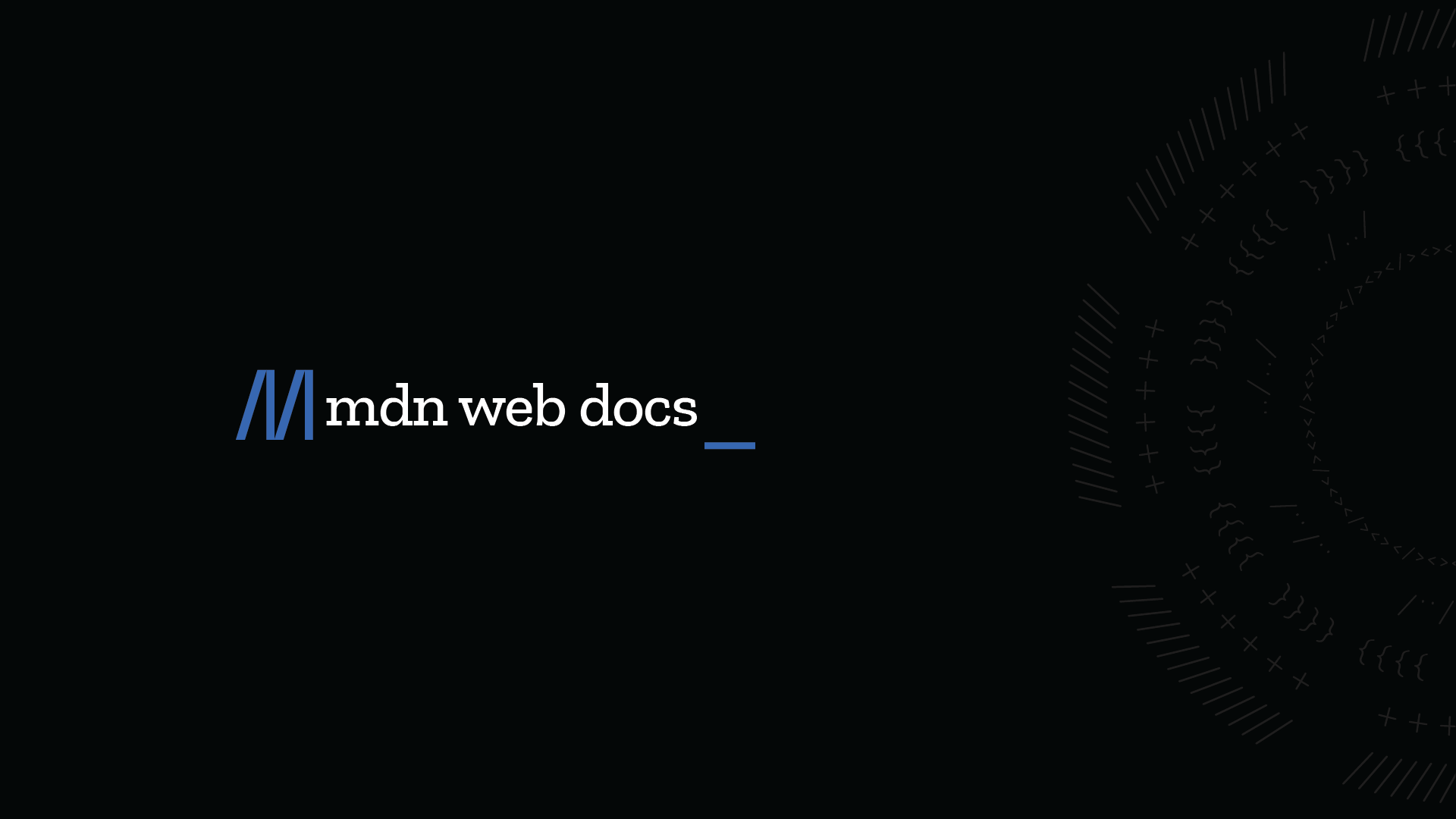
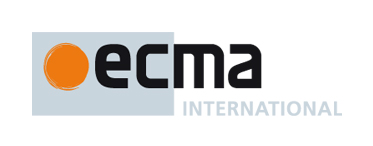
Armed with this knowledge, you’re ready to incorporate Symbols effectively into your JavaScript projects — enjoy fewer conflicts and more powerful, expressive code!
Discussion