Understanding Canvas API: A Comprehensive Guide
Canvas API enables high-performance graphics for web apps, from games to data visualizations. It offers pixel-level control, smooth animations, and custom UI components, surpassing traditional HTML elements. Mastering Canvas unlocks dynamic, interactive web experiences.
Imagine building a fast-paced online game, a real-time data dashboard, or an interactive art tool—all seamlessly rendered in the browser. The Canvas API makes this possible by providing a dynamic, high-performance way to create and manipulate graphics in web applications. Whether you're developing complex visualizations, real-time game graphics, or custom charting solutions, the Canvas API provides a way to draw and manipulate graphics efficiently using JavaScript. In this guide, we’ll explore the fundamentals of the Canvas API, its advantages over conventional HTML elements, best practices, performance optimizations, and practical use cases.
What is the Canvas API?
The Canvas API is a part of the HTML5 standard, allowing developers to create and manipulate graphics using JavaScript. It provides a bitmap-based drawing surface, making it ideal for dynamic, high-performance rendering of images, animations, charts, and game graphics. Unlike traditional HTML elements that rely on the DOM for rendering, the <canvas>
element works independently, which can significantly boost performance in applications that require frequent visual updates. This makes it particularly useful for scenarios like real-time animations and game development.
How It Works
- A
<canvas>
element is placed in the HTML document. - JavaScript is used to access its rendering context (
getContext("2d")
for 2D graphics orgetContext("webgl")
for 3D graphics). - Graphics are drawn using a variety of methods such as
fillRect()
,arc()
,lineTo()
, anddrawImage()
.
<canvas id="myCanvas" width="500" height="500"></canvas>
<script>
const canvas = document.getElementById("myCanvas");
const ctx = canvas.getContext("2d");
</script>
Why Use the Canvas API Over Conventional HTML Nodes?
HTML elements and CSS are excellent for rendering static or structured content, but they are not well-suited for performance-intensive graphics. The Canvas API offers advantages such as:
- Performance: Drawing directly onto a pixel-based surface avoids the overhead of managing DOM elements, making it much faster for dynamic rendering.
- Low-Level Control: Developers have precise control over every pixel, enabling advanced visual effects that are difficult to achieve with CSS and HTML alone.
- Game Development: The immediate rendering model is ideal for real-time updates in web games.
- Custom Graphics: Applications like image editors, physics simulations, and data visualizations benefit from the flexibility of canvas rendering.
- GPU Acceleration: The Canvas API supports WebGL, allowing hardware acceleration for better performance in 3D applications.
Best Practices for Using the Canvas API
Optimize Redrawing
- Use a single
requestAnimationFrame()
loop instead of multiple timers. - Minimize the number of draw calls by batching operations.
- Clear only necessary parts of the canvas rather than redrawing the entire scene.
ctx.clearRect(0, 0, canvas.width, canvas.height);
Use Offscreen Canvas
Rendering offscreen and transferring the image onto the main canvas improves performance by reducing the number of operations directly affecting the main canvas.
Manage Performance with Layering
- Use multiple canvases stacked in the DOM.
- Draw static elements (backgrounds) on a separate layer to prevent unnecessary re-rendering.
Handle Scaling Properly
Use canvas.width
and canvas.height
attributes instead of CSS to prevent blurriness. CSS scaling resizes the canvas visually without adjusting the actual resolution, leading to pixelation or blurriness. By setting the width and height directly, you ensure the canvas renders at the correct resolution, preserving sharpness.
const scale = window.devicePixelRatio;
canvas.width = 500 * scale;
canvas.height = 500 * scale;
ctx.scale(scale, scale);
Performance Benefits of the Canvas API
Faster Rendering
- The Canvas API operates at the pixel level, bypassing the DOM and CSS rendering pipeline.
- Ideal for applications requiring frequent updates, such as games and real-time data visualization.
Reduced Memory Overhead
- Unlike HTML elements, Canvas does not require additional DOM nodes.
- No reflow or repaint issues from complex nested elements.
GPU Acceleration
- Supports WebGL (
getContext('webgl')
), leveraging hardware acceleration for improved performance.
Use Cases for the Canvas API
Game Development
- Real-time rendering for 2D and 3D games.
- Uses
requestAnimationFrame()
for smooth animations.
Data Visualization & Charts
- Libraries like Chart.js and D3.js utilize canvas for efficient graph rendering.
- Allows dynamic updates without impacting page performance.
Image Manipulation & Editing
- Enables filters, transformations, and pixel-based effects.
- Used in online photo editors like Pixlr.
Video Effects & Streaming
- Can process real-time video data and apply filters.
- Example: Drawing frames from a video onto a canvas.
ctx.drawImage(videoElement, 0, 0, canvas.width, canvas.height);
Custom UI Components
- Used for interactive signature pads and non-standard UI elements.
- Enables dynamic rendering without relying on static HTML elements.
Why Use Canvas Instead of HTML Nodes?
Feature | Canvas API | HTML (DOM) |
---|---|---|
Performance | Faster, optimized for rendering | Slower, reflow/repaint issues |
Interactivity | Requires manual event handling | Native event listeners |
Scalability | Great for high-frequency updates | Complex DOM structures become heavy |
Complexity | Procedural drawing, requires JavaScript | Declarative, easier for static elements |
GPU Usage | Supports WebGL acceleration | Limited hardware acceleration |
Use Canvas when:
- You need real-time rendering.
- Performance is critical (games, visualizations).
- You are building custom, non-standard UI elements.
Use HTML/CSS/SVG when:
- You need accessibility and SEO benefits.
- The UI is mostly static.
- You require built-in event handling.
Common Challenges & Solutions
Accessibility Issues
- Canvas does not have built-in accessibility features like HTML.
- Provide alternative text descriptions and use ARIA attributes for interactive elements.
State Management
- Canvas is stateless; once something is drawn, it stays until manually erased.
- Maintain an offscreen object model and use a repaint loop to redraw necessary changes.
Debugging & Readability
- Use modular functions to structure drawing logic.
- Employ object-oriented programming for interactive elements.
The Future of Canvas API
- WebGPU: A next-generation graphics API for better GPU performance.
- OffscreenCanvas: Allows rendering in web workers to avoid blocking the main thread.
- CanvasKit & Skia: High-performance rendering engines used by Google.
Additional Resources
For further learning and practical demonstrations, here are some valuable resources related to the Canvas API:
Official Documentation
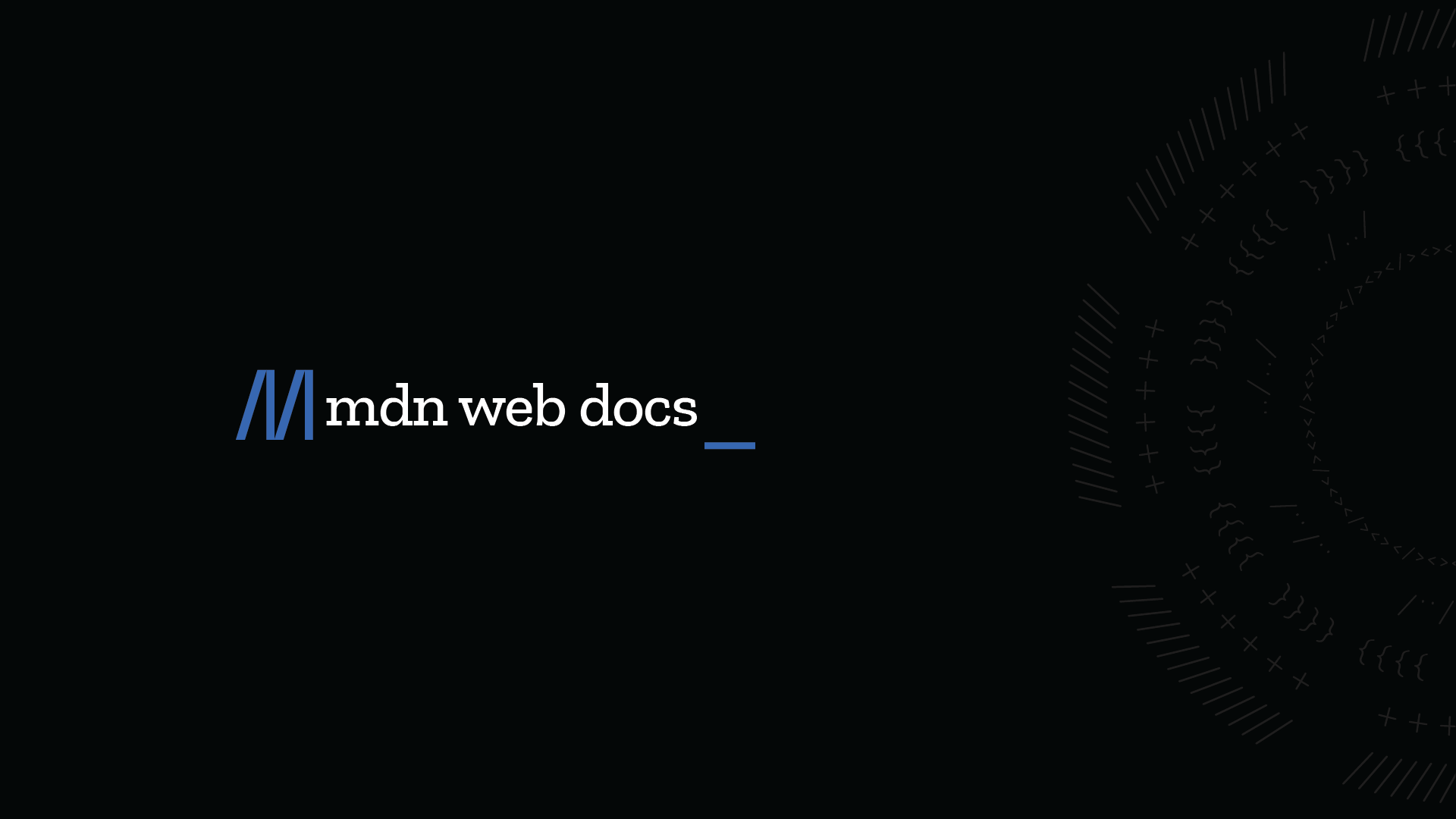
Practical Demos
Game Development
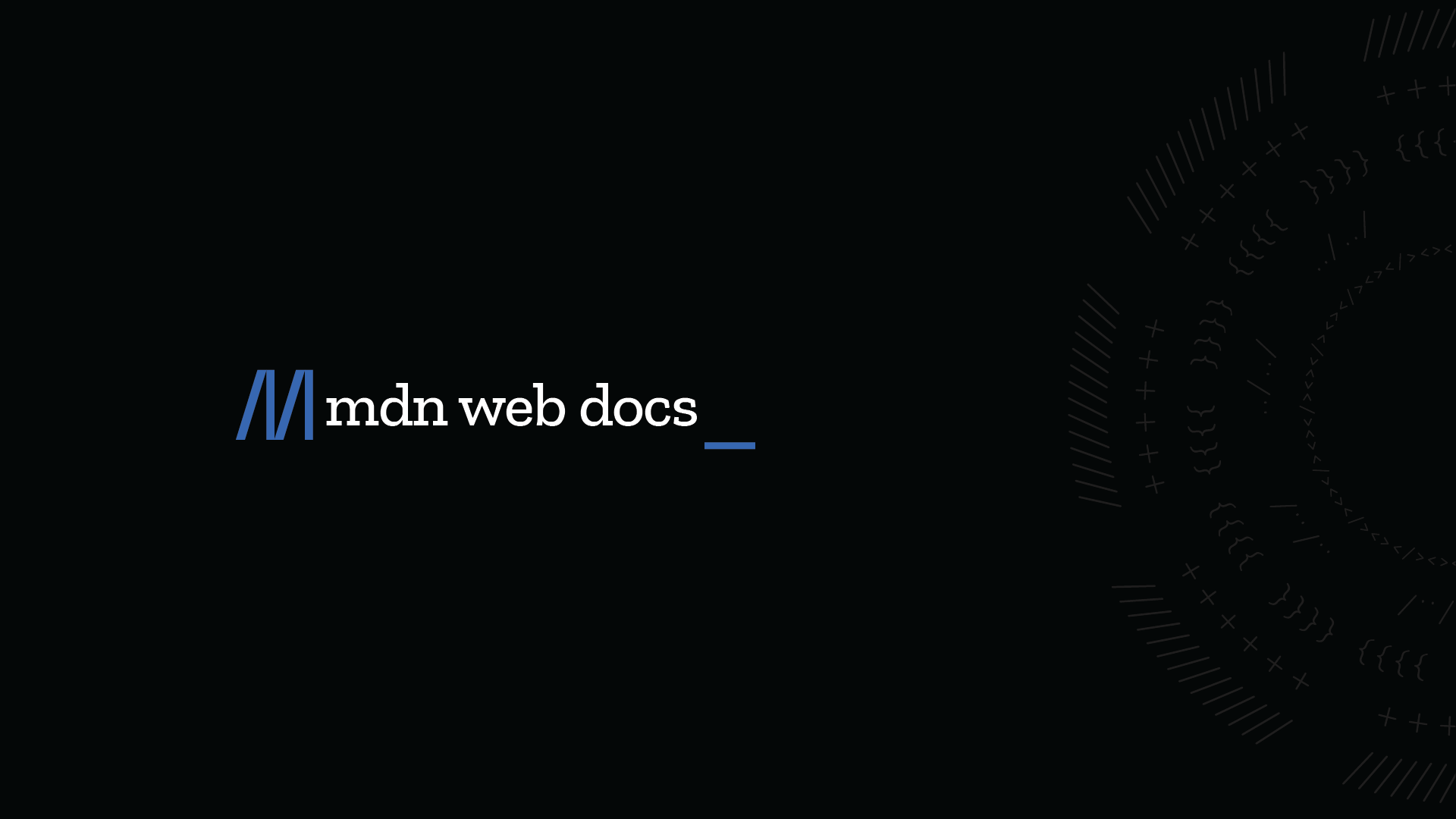

Data Visualization & Charts
Image Manipulation & Editing
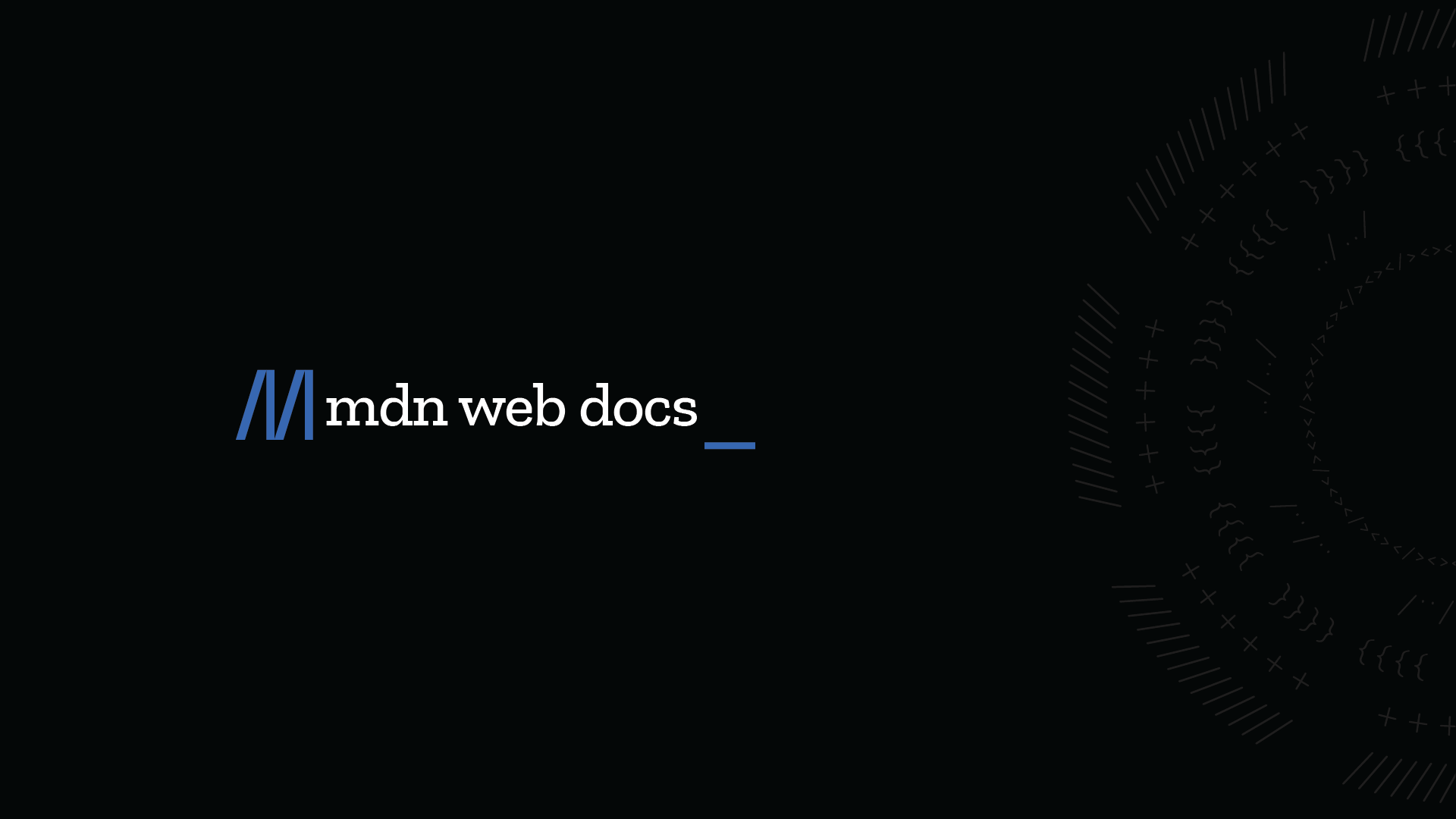
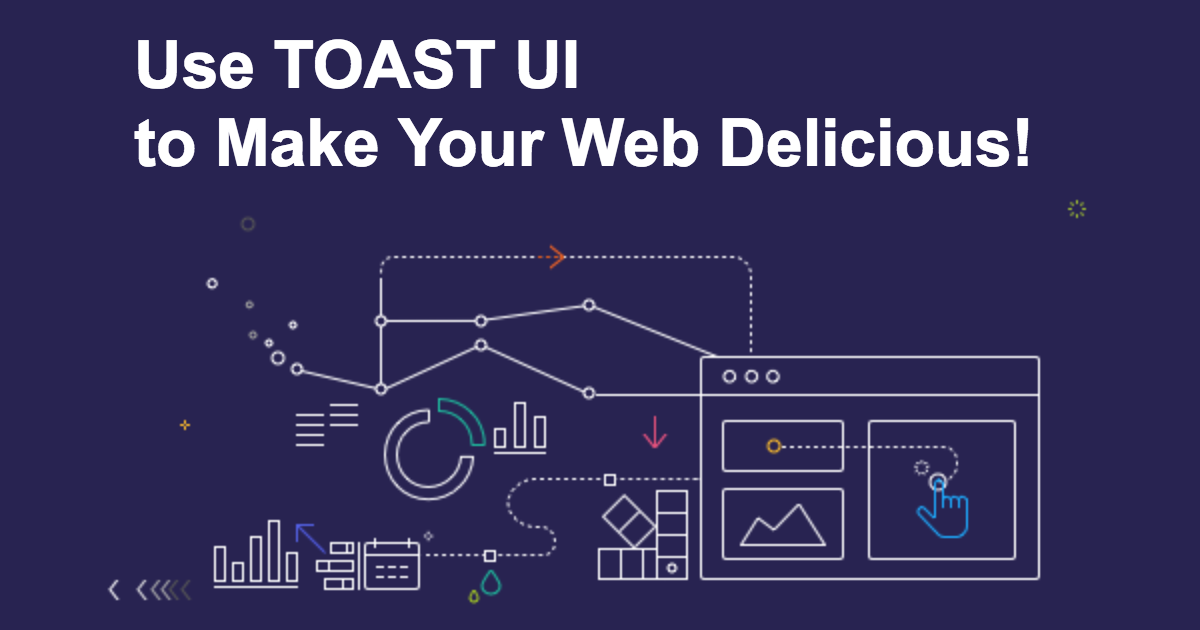
Video Effects & Streaming
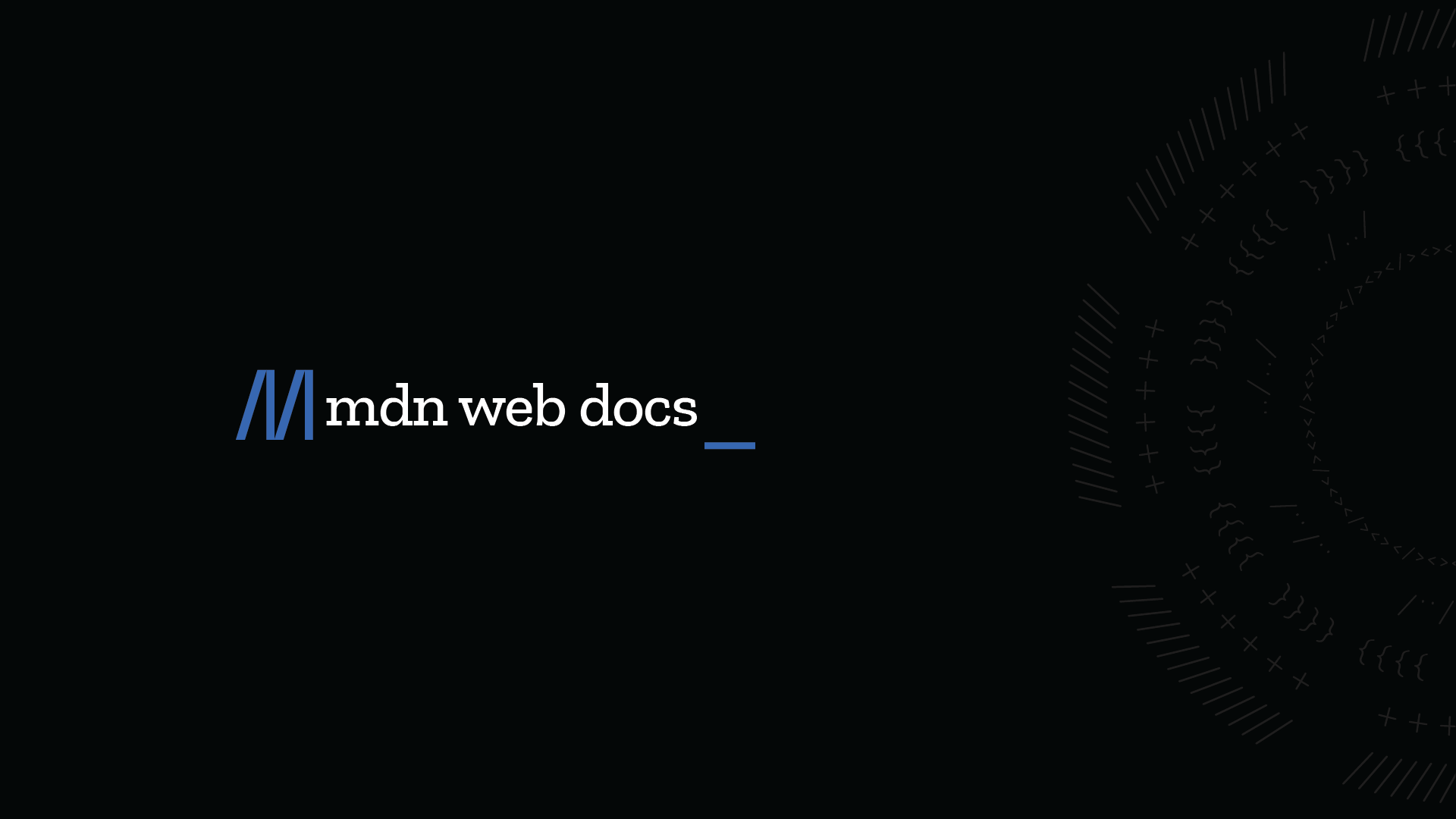
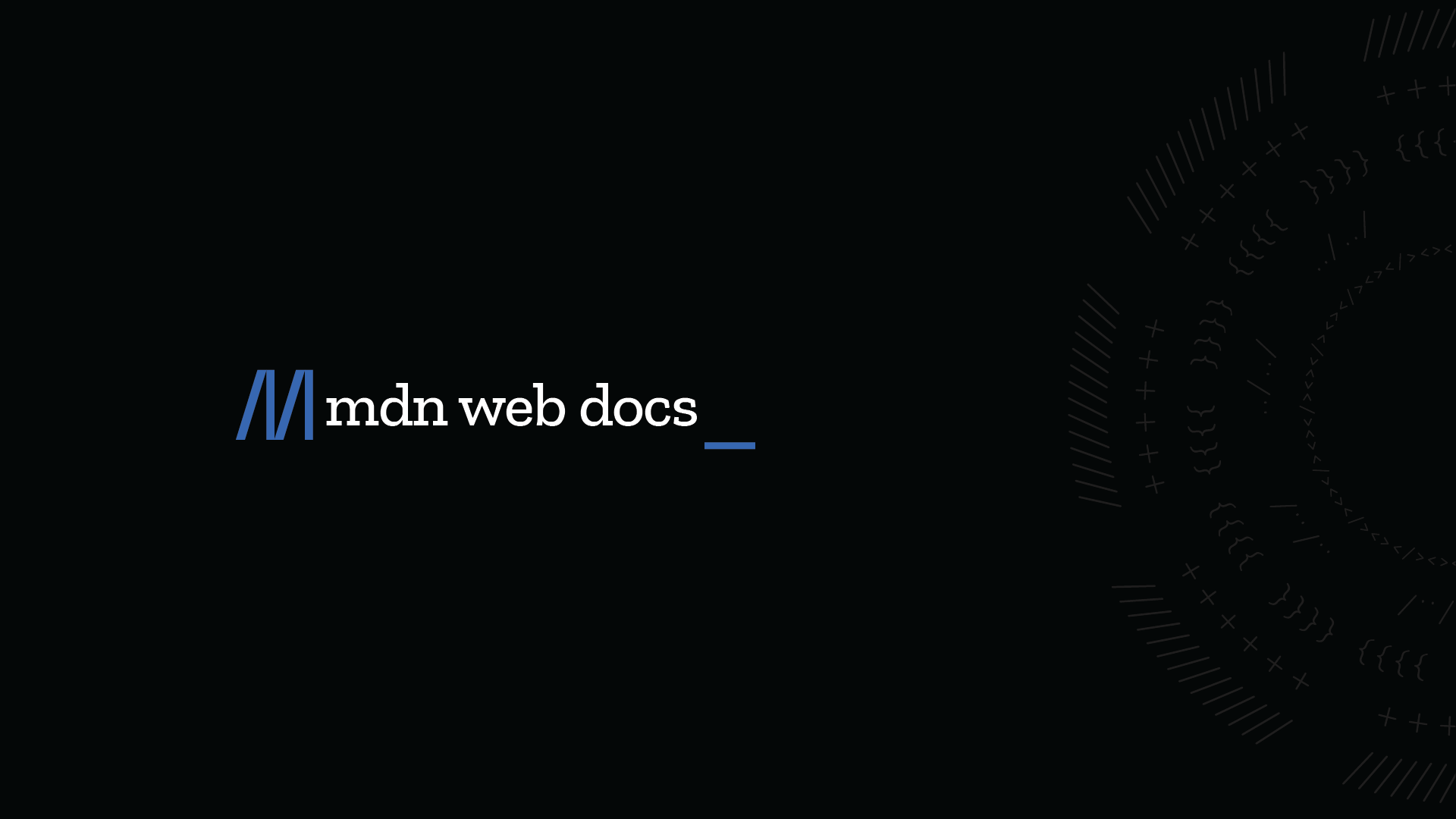
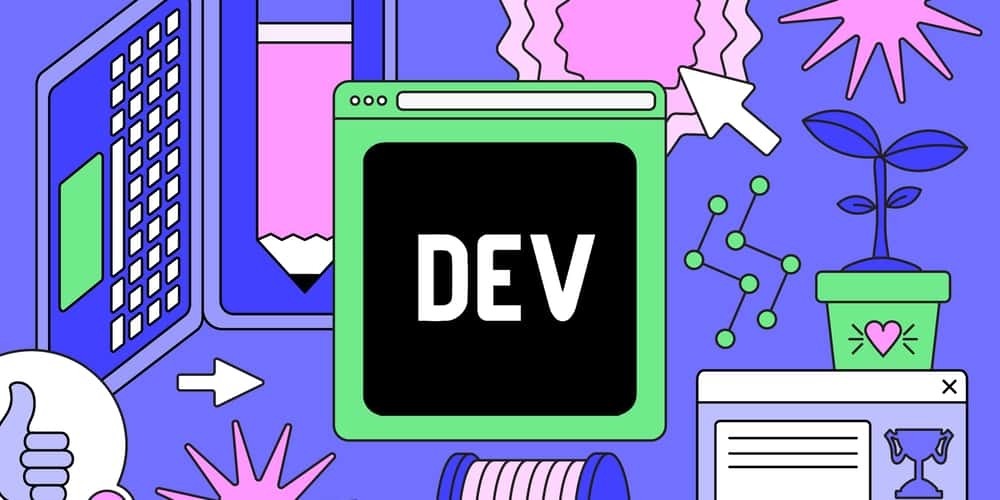
Custom UI Components
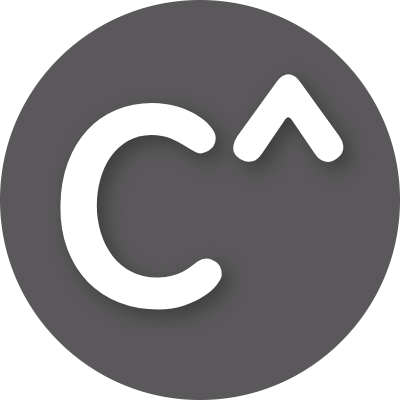
Conclusion
The Canvas API is an essential tool for high-performance, graphics-intensive applications. As web technologies continue to evolve, Canvas remains a powerful choice for creating immersive, interactive experiences. By mastering its capabilities, developers can push the boundaries of web graphics and bring their creative visions to life. By following best practices, optimizing performance, and leveraging modern GPU-powered enhancements, developers can maximize the potential of Canvas for efficient and dynamic web applications.
Discussion