Preventing AI-Caused Tech Debt: How to Enforce Clean Architecture from Day One
AI tools accelerate development—but often at the cost of architectural integrity. This guide shows how to prevent AI-induced tech debt by enforcing Clean Architecture from day one using automation, static analysis, and AI-readable documentation.
Introduction
AI-assisted coding is no longer on the horizon—it’s already reshaping software development workflows. Tools like GitHub Copilot, ChatGPT, and IDE-integrated agents such as Cursor and Windsurf are writing code, scaffolding projects, and even generating architectural suggestions. What began as autocomplete-on-steroids has evolved into an always-on co-developer embedded in the IDE. These tools are revolutionizing velocity—but not without cost.
For all their power, AI code generators introduce a subtle yet dangerous risk: architectural erosion. Unlike human developers, AI tools lack an innate understanding of your system’s boundaries, domain rules, or layering strategies. They optimize for immediacy—solving the problem at hand—rather than long-term cohesion. When AI-generated code goes unchecked, it often bypasses established architectural norms, leaks concerns across layers, and accelerates the accrual of technical debt.
This risk isn't theoretical. In AI-first teams, it’s increasingly common to find infrastructure logic embedded in business services, domain logic entangled with UI layers, and new modules shaped more by prompt phrasing than architectural intent. What’s more, traditional guardrails—manual code reviews and pair programming—struggle to keep pace with AI’s output. The result is a quiet drift away from maintainability and toward entropy.
So how do we prevent this?
This article proposes a three-pronged approach: use Clean Architecture as a foundational blueprint, enforce it through automation from day one, and treat developer-facing documentation not as a static artifact but as a dynamic contract—one legible to both humans and AI. By establishing architectural constraints early, codifying them in tooling, and expressing them in machine-readable documentation, teams can build a system of safeguards that scales with AI-accelerated development.
We’ll explore:
- The specific architectural anti-patterns introduced by AI-generated code.
- How to bootstrap projects with Clean Architecture principles.
- The role of linters, static analysis, and CI pipelines in enforcement.
- How to gate AI contributions and guide AI agents with structured documentation.
This is not about fighting AI. It’s about designing development ecosystems where AI works within boundaries—not outside them.
Are you ready to take control from day one?
The Nature of AI-Induced Tech Debt
AI code generation is a productivity accelerant—but like all accelerants, it can scorch the foundations if left unmanaged. To understand how this happens, we need to examine the types of architectural debt that AI tools most commonly introduce. These are not bugs in the traditional sense. They're structural flaws: violations of layering, misplaced responsibilities, and shortcuts that weaken system cohesion over time.
Common AI-Induced Anti-Patterns
Let’s walk through the most prevalent architectural issues introduced by AI-generated code, with concrete TypeScript examples.
1. Leaking Infrastructure into the Domain Layer
Clean Architecture demands that domain logic remain independent of frameworks and infrastructure. AI, unaware of these rules, often injects database or HTTP logic directly into domain services.
Problematic Example – A domain service that directly calls a database client:
// domain/services/user-service.ts
import { db } from "../infrastructure/db"; // BAD: infrastructure leak
export class UserService {
async getUserProfile(userId: string) {
const user = await db.user.findUnique({ where: { id: userId } });
return { name: user?.name, email: user?.email };
}
}
This breaks the dependency rule: domain should not depend on infrastructure. Over time, this kind of logic fragments the boundary between business logic and persistence.
Refactored Example – Use an interface to decouple domain from infrastructure:
typescriptCopyEdit
// domain/ports/user-repository.ts
export interface UserRepository {
findById(id: string): Promise<{ name: string; email: string } | null>;
}
// domain/services/user-service.ts
import { UserRepository } from "../ports/user-repository";
export class UserService {
constructor(private readonly repo: UserRepository) {}
async getUserProfile(userId: string) {
return this.repo.findById(userId);
}
}
// infrastructure/repositories/prisma-user-repository.ts
import { UserRepository } from "../../domain/ports/user-repository";
import { db } from "../db";
export class PrismaUserRepository implements UserRepository {
async findById(id: string) {
const user = await db.user.findUnique({ where: { id } });
return user ? { name: user.name, email: user.email } : null;
}
}
2. Skipping Layered Boundaries Entirely
AI-generated code often shortcuts layering. It may generate an endpoint that talks directly to the database, bypassing the service and domain layers altogether.
Problematic Example – Route handler accessing persistence directly:
// routes/user-routes.ts
import express from "express";
import { db } from "../infrastructure/db";
const router = express.Router();
router.get("/user/:id", async (req, res) => {
const user = await db.user.findUnique({ where: { id: req.params.id } });
res.json(user);
});
This seems convenient but makes the system brittle and hard to refactor.
Refactored Example – Route delegates to an application layer use case:
// application/useCases/get-user-profile.ts
import { UserService } from "../../domain/services/user-service";
export class GetUserProfile {
constructor(private readonly service: UserService) {}
async execute(id: string) {
return this.service.getUserProfile(id);
}
}
// routes/userRoutes.ts
import express from "express";
import { PrismaUserRepository } from "../infrastructure/repositories/prisma-user-repository";
import { UserService } from "../domain/services/user-service";
import { GetUserProfile } from "../application/useCases/get-user-profile";
const router = express.Router();
const userRepo = new PrismaUserRepository();
const userService = new UserService(userRepo);
const getUserProfile = new GetUserProfile(userService);
router.get("/user/:id", async (req, res) => {
const user = await getUserProfile.execute(req.params.id);
res.json(user);
});
3. Domain Logic Embedded in Controllers
Controllers (or route handlers) should be thin—just translating HTTP to application calls. But AI often embeds conditional logic, validation, or orchestration directly into controllers.
Problematic Example:
router.post("/register", async (req, res) => {
const { email, password } = req.body;
if (!email.includes("@")) {
return res.status(400).json({ error: "Invalid email" });
}
const existingUser = await db.user.findUnique({ where: { email } });
if (existingUser) {
return res.status(400).json({ error: "User already exists" });
}
await db.user.create({ data: { email, password } });
res.status(201).send("User created");
});
Refactored Example – Push all decisions into the domain/application layer:
// domain/services/user-service.ts
export class UserService {
async registerUser(email: string, password: string) {
if (!email.includes("@")) throw new Error("Invalid email");
const user = await this.repo.findByEmail(email);
if (user) throw new Error("User already exists");
await this.repo.create({ email, password });
}
}
Why Manual Review Fails at Scale
Historically, these problems were caught in code review. But AI-generated code changes the dynamics:
- AI can generate dozens of file edits in minutes.
- Much of the code is syntactically correct and passes tests.
- Reviewers assume AI-generated logic is “smart,” not realizing it lacks context.
- Review fatigue is real—especially when dealing with boilerplate or repetitive patterns.
Manual enforcement simply doesn’t scale. What teams need is automated governance—rules and signals that prevent violations from ever reaching the main branch.
Clean Architecture from Day One
AI doesn’t forget—but it also doesn’t understand. That’s why relying on AI to “just follow best practices” isn’t enough. Without guardrails, AI-generated code will default to patterns that appear to work in isolation, but ignore your system’s architectural vision. The best defense? Establish that vision explicitly from the very first line of code.
Clean Architecture provides an ideal starting point: it’s modular, testable, and adaptable. More importantly, it’s enforceable—with clear boundaries that AI can be guided (or constrained) to follow.
What Is Clean Architecture?
Clean Architecture, popularized by Robert C. Martin (“Uncle Bob”), organizes code into concentric circles or layers. The deeper a layer, the more abstract and stable it is.
Here’s a breakdown of the typical layers:
- Entities (Domain Models): Core business logic and rules.
- Use Cases (Application Services): Coordinate operations, orchestrate domain logic.
- Interfaces (Adapters): Gateways like HTTP handlers, CLI, or GraphQL resolvers.
- Infrastructure: Frameworks, databases, external services.
The key rule: Dependencies point inward. No outer layer should “know” about inner layers beyond abstract contracts.
Visual Diagram – Clean Architecture in TypeScript (simplified)
+----------------------------+
| Interfaces | (Express routes, CLI, GraphQL)
+----------------------------+
| Use Cases | (Application logic, services)
+----------------------------+
| Domain Models | (Business rules, value objects)
+----------------------------+
| Infrastructure | (DB, frameworks, APIs) ← depends on domain contracts
+----------------------------+
Why Start From Day One?
Most architecture failures aren't because teams didn't know what “Clean Architecture” was—they just didn't commit to it early enough. Once a project grows past a dozen modules and hundreds of commits, retrofitting architectural boundaries becomes costly and contentious.
By contrast, establishing those boundaries up front allows you to:
- Guide AI and human contributors with consistent patterns.
- Prevent coupling between domain logic and infrastructure.
- Scale features without turning the codebase into spaghetti.
Let’s look at how to bootstrap this effectively in a TypeScript project.
Bootstrapping a Clean Architecture Project in TypeScript
Here’s a recommended folder structure and separation strategy from day one:
/src
/domain
/models ← Entities, value objects
/ports ← Interfaces (e.g., IUserRepository)
/services ← Pure domain logic
/application
/usecases ← Business workflows, orchestration
/infrastructure
/db ← Database clients (e.g., Prisma)
/repositories ← Adapter implementations
/http ← Express/Koa adapters
/interfaces
/routes ← Express routes or controllers
/shared ← Cross-cutting utils, types
Example: Creating a Domain-Centric Feature
Let’s build a simple “user registration” feature using Clean Architecture principles from the start.
1. Define the domain model
// domain/models/user.ts
export class User {
constructor(
public readonly id: string,
public readonly email: string,
public readonly hashedPassword: string
) {}
static isValidEmail(email: string): boolean {
return email.includes("@");
}
}
2. Define a domain interface (port)
// domain/ports/user-repository.ts
export interface UserRepository {
findByEmail(email: string): Promise<User | null>;
save(user: User): Promise<void>;
}
3. Write pure domain logic
// domain/services/user-service.ts
import { User } from "../models/user";
import { UserRepository } from "../ports/user-repository";
export class UserService {
constructor(private readonly repo: UserRepository) {}
async registerUser(email: string, password: string): Promise<User> {
if (!User.isValidEmail(email)) {
throw new Error("Invalid email");
}
const existing = await this.repo.findByEmail(email);
if (existing) throw new Error("Email already registered");
const hashed = await this.hashPassword(password); // pretend this is pure
const user = new User(this.generateId(), email, hashed);
await this.repo.save(user);
return user;
}
private async hashPassword(pw: string) {
// Hashing logic abstracted for clarity
return `hashed-${pw}`;
}
private generateId() {
return crypto.randomUUID();
}
}
4. Implement the repository
// infrastructure/repositories/prisma-user-repository.ts
import { UserRepository } from "../../domain/ports/user-repository";
import { User } from "../../domain/models/user";
import { db } from "../db";
export class PrismaUserRepository implements UserRepository {
async findByEmail(email: string): Promise<User | null> {
const result = await db.user.findUnique({ where: { email } });
if (!result) return null;
return new User(result.id, result.email, result.hashedPassword);
}
async save(user: User): Promise<void> {
await db.user.create({
data: {
id: user.id,
email: user.email,
hashedPassword: user.hashedPassword,
},
});
}
}
5. Application use case
// application/usecases/RegisterUser.ts
import { UserService } from "../../domain/services/user-service";
export class RegisterUser {
constructor(private readonly service: UserService) {}
async execute(email: string, password: string) {
return this.service.registerUser(email, password);
}
}
6. Route handler
// interfaces/routes/user-routes.ts
import express from "express";
import { PrismaUserRepository } from "../../infrastructure/repositories/prisma-user-repository";
import { UserService } from "../../domain/services/user-service";
import { RegisterUser } from "../../application/usecases/register-user";
const router = express.Router();
const repo = new PrismaUserRepository();
const service = new UserService(repo);
const registerUser = new RegisterUser(service);
router.post("/register", async (req, res) => {
const { email, password } = req.body;
try {
const user = await registerUser.execute(email, password);
res.status(201).json(user);
} catch (err: any) {
res.status(400).json({ error: err.message });
}
});
Architectural Benefits
By establishing this structure from the outset:
- AI contributions can be gated to fit the existing architecture (e.g., new use cases follow the same
usecases/service/repo
flow). - It’s easier to enforce boundaries via tooling (which we’ll cover in the next section).
- New developers—and AI agents—have a clear map of where code belongs.
Enforcement Through Linters & Static Analysis
Establishing clean architecture is step one. But maintaining it—especially in an AI-augmented workflow—requires enforcement. Without automated boundaries, your clean architecture will erode line by line as developers (and AI) introduce “just this once” shortcuts.
Linters and static analysis tools act as your automated code guardians. They don’t just flag formatting issues—they can enforce file boundaries, layering conventions, dependency direction, and even architectural rules. With proper configuration, these tools can block violations before code ever reaches the main branch.
Let’s walk through how to build an automated enforcement system for TypeScript projects.
Enforcing Code & Architecture with Linters
The TypeScript ecosystem has powerful tools to enforce both code quality and architectural intent. Let’s examine the most relevant ones.
ESLint (with Custom Rules for Layering)
ESLint is the standard linter for TypeScript and JavaScript, but its power extends beyond catching semicolons and unused vars. With plugins like eslint-plugin-boundaries
, you can enforce architectural layering directly.
Installation:
bashCopyEditnpm install --save-dev eslint eslint-plugin-boundaries
Example .eslintrc.js
configuration:
module.exports = {
plugins: ["boundaries"],
rules: {
"boundaries/element-types": [2, {
default: "disallow",
rules: [
{
from: "interfaces",
allow: ["application"]
},
{
from: "application",
allow: ["domain"]
},
{
from: "domain",
allow: [] // Domain should depend on nothing
},
]
}],
},
settings: {
"boundaries/elements": [
{ type: "interfaces", pattern: "src/interfaces/*" },
{ type: "application", pattern: "src/application/*" },
{ type: "domain", pattern: "src/domain/*" },
{ type: "infrastructure", pattern: "src/infrastructure/*" },
]
}
}
Example Violation (flagged):
// src/domain/services/user-service.ts
import { db } from "../../infrastructure/db"; // ❌ ESLint boundary rule violation
This prevents domain logic from accessing infrastructure directly.
Modular Dependency Rules with depcheck
or madge
For visual and static analysis of dependencies, madge
can render import graphs and detect circular or invalid dependencies.
Install and run:
npm install --save-dev madge
madge --circular --extensions ts src/
madge --image graph.svg src/
This allows you to detect architectural violations at a glance and integrate this check into CI.
Custom Static Analysis with ts-morph
When linters aren’t enough, ts-morph
allows deep inspection of TypeScript ASTs (Abstract Syntax Trees), enabling custom scripts to enforce project-specific architecture.
Example Use Case: Flag domain files that import anything from /infrastructure/
.
import { Project } from "ts-morph";
const project = new Project();
project.addSourceFilesAtPaths("src/domain/**/*.ts");
const violations = [];
for (const sourceFile of project.getSourceFiles()) {
const imports = sourceFile.getImportDeclarations();
for (const imp of imports) {
const module = imp.getModuleSpecifierValue();
if (module.includes("/infrastructure/")) {
violations.push({
file: sourceFile.getFilePath(),
import: module
});
}
}
}
if (violations.length) {
console.error("Architecture violations detected:");
console.table(violations);
process.exit(1); // CI can fail here
}
You can plug this into your CI pipeline to ensure zero violations reach production.
Using SonarQube for Higher-Level Analysis
SonarQube is a powerful static analysis platform that can:
- Detect architectural drift
- Monitor code smells, complexity, and duplication
- Enforce quality gates before merges
CI/CD Integration Example (GitHub Actions):
jobs:
sonar:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: SonarQube Scan
uses: SonarSource/sonarqube-scan-action@master
env:
SONAR_TOKEN: ${{ secrets.SONAR_TOKEN }}
with:
projectBaseDir: .
This ensures architectural rules are applied at the organizational level—not just individual projects.
CI Pipeline Enforcement
Here’s how you can structure a GitHub Actions CI pipeline to gate AI-generated or human code from violating architectural rules:
name: Lint & Architecture Check
on: [push, pull_request]
jobs:
lint:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Setup Node.js
uses: actions/setup-node@v3
with:
node-version: 18
- name: Install dependencies
run: npm ci
- name: Run ESLint
run: npm run lint
- name: Run Custom Architecture Check
run: node scripts/architecture-check.js
- name: Check Dependency Graph
run: npx madge --circular src/
Bonus: Gating Lint Failures in VS Code or Cursor
To guide AI-assisted IDEs (like Cursor), you can use .editorconfig
, preconfigured ESLint settings, and workspace rules to reject or flag generated suggestions that violate architectural norms in real-time.
By embedding these tools into your development lifecycle, you shift enforcement left—closer to the developer (or AI) and further from production. These aren’t just best practices—they’re non-negotiable contracts encoded in your tooling.
Gating AI-Generated Code & Documentation as Architectural Contracts
The challenge with AI-generated code isn’t just quality—it’s context blindness. Large language models don’t “understand” your project architecture; they predict code based on patterns in training data or inline context. Without guardrails, they’ll inject data access into route handlers, skip abstractions, and violate dependency rules.
So how do we gate AI-generated code contributions before they rot our architecture?
This section is divided into two parts:
- A. Gating AI-Generated Code with policies, bots, and tooling.
- B. Documentation as Enforcer & Signal for AI IDEs—a future-ready practice.
A. Gating AI-Generated Code
While you can’t control how AI writes code in the developer’s IDE, you can control what gets merged. Here’s how to intercept and reject architecture-violating AI code before it hits your main branch.
Use Pre-Commit Hooks to Catch Violations Early
Tools like Husky and lint-staged allow you to block commits that break rules—before they’re even staged.
Install Husky:
bashCopyEditnpx husky-init && npm install
Example Pre-Commit Hook (.husky/pre-commit
):
#!/bin/sh
. "$(dirname "$0")/_/husky.sh"
npm run lint
node scripts/architecture-check.js
Result: If a developer—or AI—tries to commit code that violates layering, the commit fails locally.
Use GitHub Actions to Gate PRs from AI IDEs
Cursor, GitHub Copilot, and other AI tools often operate in agentic modes—generating and committing code on the developer’s behalf. GitHub Actions can detect these PRs and apply additional scrutiny.
Step 1: Label AI-generated commits
Instruct developers to tag PRs with [ai]
, or use commit heuristics (e.g., authored-by: cursor
).
Step 2: Write a GitHub Action that blocks merge on policy violations
name: Enforce AI Contribution Policy
on: pull_request
jobs:
enforce-architecture:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Run Architectural Checks
run: |
npm ci
npm run lint
node scripts/architecture-check.js
- name: Block Merge if AI-generated
if: contains(github.event.pull_request.title, '[ai]')
run: |
echo "AI-generated PR detected. Manual review required."
exit 1
This fails the build for PRs marked [ai]
unless they pass architectural checks.
Add a Bot Reviewer for Architecture Violation Detection
Using Danger.js, you can build a bot that leaves comments on PRs if they violate architectural contracts.
Example Dangerfile (in TypeScript):
import { schedule, fail, warn } from "danger";
schedule(async () => {
const modifiedFiles = danger.git.modified_files;
const domainViolations = modifiedFiles.filter(file =>
file.includes("domain/") && file.includes("infrastructure/")
);
if (domainViolations.length > 0) {
fail("⚠️ Domain layer is importing infrastructure. Violates Clean Architecture.");
}
});
Run it in CI to catch violations and notify reviewers automatically.
B. Documentation as Enforcer & Signal for AI IDEs
Now comes the emerging best practice: using documentation not just for humans, but as a structured signal for AI-powered IDEs like Cursor and Windsurf.
These tools already analyze your codebase to generate inline suggestions. By augmenting your docs with clear architectural intent, you guide AI to respect boundaries rather than guess them.
What Is “AI-Readable Documentation”?
AI-readable documentation isn’t about prose. It’s about structure, metadata, and architectural clarity. Think of it as a way to teach the AI your architecture.
Tactics for Writing Documentation AI Can Use
1. Structured README Files per Layer
Each module or layer (e.g., domain/
, application/
, infrastructure/
) should include a README.md
with:
- Purpose of the module.
- What it is allowed to import or be used by.
- What it must not contain.
Example src/domain/README.md
:
# Domain Layer
- Pure business logic: entities, value objects, interfaces.
- No dependencies on infrastructure, databases, or HTTP.
- Only consumed by the `application/` layer.
This layer should not:
- Import from `infrastructure/`
- Handle requests or responses
- Use framework-specific code
Architecture Rule:
- Depends on nothing. Only exports contracts.
2. Inline Comments as AI Signals
AI agents like Cursor analyze surrounding comments. Use comments to encode layering rules.
// application/usecases/RegisterUser.ts
// Application layer. Only interacts with domain services and ports.
// Do not include HTTP, database, or third-party logic here.
3. Module-Level Metadata Files
Some teams use module.json
or similar files to describe layering and allowed imports.
Example domain/module.json
:
{
"layer": "domain",
"allowedToDependOn": [],
"exportedInterfaces": ["User", "UserRepository"]
}
AI agents can be trained or configured to interpret these during suggestion generation.
Use ADRs to Lock in Architectural Decisions
Architectural Decision Records (ADRs) are markdown files that track architectural choices over time.
Example: docs/adr/001-layering.md
# ADR-001: Enforce Clean Layering
Context:
We are adopting Clean Architecture to isolate domain logic.
Decision:
- Domain → no dependencies.
- Application → uses domain only.
- Interfaces → uses application.
- Infrastructure → implements ports from domain.
Status: Approved
Consequences:
Violations will be flagged via ESLint and CI policies.
These can also be referenced in pull requests to guide contributors (and AI reviewers).
Keep Documentation Synchronized
Use tools like:
- Doclint: Lint markdown and enforce structure.
- documentation-as-code: Keep docs version-controlled and reviewed.
- Typedoc: Generate API docs from TypeScript interfaces for AI context.
You can even include documentation lints in CI:
- name: Lint Docs
run: npx markdownlint-cli2 docs/**/*.md
Together, automation and documentation form a closed loop:
- Tooling enforces structure.
- Documentation explains and teaches the structure.
- AI learns and works within that structure.
Best Practices and Pitfalls
Enforcing Clean Architecture in an AI-enhanced codebase isn’t just about rules and tools—it’s about building sustainable developer habits. Even the best-automated safeguards can be circumvented by unclear intentions, prompt misuse, or poorly aligned team expectations. In this section, we’ll look at common mistakes and proven best practices from teams navigating the frontier of AI-assisted software development.
Common Pitfalls in AI-Driven Codebases
Even with CI, linters, and documented architecture in place, technical debt creeps in if teams fall into these traps:
1. Assuming AI “Understands” Your Architecture
This is the foundational mistake. AI tools like Copilot or Cursor don’t inherently understand your business layers, dependency rules, or module boundaries. They follow syntax and statistical inference—not your design patterns.
Symptoms:
- AI-generated services using
prisma
directly in domain code. - Route handlers that skip use cases and manipulate models directly.
- DTOs duplicated across multiple layers.
Fix:
Guide AI with structured docs, curated examples, and strict linters that catch violations early.
2. Overreliance on Prompt Engineering
Some teams try to "hack" AI behavior by embedding architectural instructions into prompts:
“Write a service that follows Clean Architecture, separates concerns, and doesn’t touch infrastructure.”
While helpful, this is not a safeguard. Prompt intent can easily be overridden by autocomplete suggestions, partial context, or developer tweaks.
Fix:
Prompt responsibly—but reinforce with codebase constraints and architectural scaffolding. Treat prompts as input, not policy.
3. Skipping the Bootstrapping Step
Many teams generate code first and organize it later. This works for CRUD demos, but at scale leads to untestable, fragile systems. AI accelerates this entropy by generating large volumes of code quickly.
Fix:
Prioritize architecture before functionality. Bootstrap with domain models, use cases, and layering from the first feature. Use generators or templates (e.g., Yeoman, Plop) to scaffold features into the right place.
4. Neglecting Tool Configuration
Linters and static analysis tools are only as good as their config. Generic ESLint rules catch stylistic issues—not architecture. If your eslintrc.js
doesn't enforce module boundaries, you’re not preventing erosion.
Fix:
Invest in custom rules. Use eslint-plugin-boundaries
, madge
, and ts-morph
scripts. Review these configs like production code.
5. Letting Documentation Go Stale
AI models like Cursor’s inline copilots learn from your comments and documentation. If your docs fall out of sync, the AI’s suggestions will too.
Fix:
- Treat documentation as a version-controlled contract.
- Use CI to lint and validate markdown.
- Assign architectural documentation ownership to a lead or rotating role.
Best Practices That Actually Work
Let’s pivot to success patterns—what high-performing AI-native teams are doing right.
1. Create a Playbook for AI Contributions
Instead of ad hoc AI use, define workflows.
Sample Playbook Excerpt:
Use Cursor or Copilot to generate internal logic, not full files.Always scaffold features usingplop generate feature
.UseREADME.md
in each layer to remind AI and devs of architectural rules.Runnpm run lint:arch
before every commit.Never importdb
or third-party APIs in/domain
.
Document this in your repo's CONTRIBUTING.md
and reference it in PR templates.
2. Use Templates and Generators
Tools like Plop or Hygen let you codify how to scaffold new features, enforcing directory placement and boilerplate alignment.
Example Plop generator:
module.exports = function (plop) {
plop.setGenerator("usecase", {
description: "Generate a new use case",
prompts: [{ type: "input", name: "name", message: "Use case name" }],
actions: [
{
type: "add",
path: "src/application/usecases/{{pascalCase name}}.ts",
templateFile: "plop-templates/usecase.hbs",
},
],
});
}
This ensures new code starts in the right layer with the right abstraction.
3. Define Merge Gates by Layer
Make it harder to merge architectural violations than to fix them.
Examples:
- PRs touching
domain/
require review from senior engineer. - PRs from
[ai]
or Copilot triggers must pass full architectural CI. - Merges to
main
require all boundary checks to pass.
You can enforce this using GitHub CODEOWNERS and status checks.
4. Refactor Prompt-by-Prompt
Teach AI to conform by guiding it one prompt at a time. If Cursor generates an infrastructure-coupled service, don’t just delete it—rewrite the prompt and correct the example. Over time, this trains your workspace context and reduces the recurrence of architectural violations.
5. Run Architectural CI Locally
To avoid wasted PR cycles, give developers fast feedback locally.
Add to package.json
:
"scripts": {
"lint:arch": "node scripts/architecture-check.js",
"check": "npm run lint && npm run lint:arch && npm test"
}
Now developers (and AI agents) can verify architecture in seconds.
Summary of Best Practices
Principle | Practice |
---|---|
Define architecture early | Bootstrap with clean layers and strict boundaries |
Enforce in tooling | Use ESLint, ts-morph , madge , and CI workflows |
Gate AI contributions | Label, lint, and block merge for architecture violations |
Teach via documentation | Structured README.md , ADRs, module contracts |
Treat prompts as suggestions | Always review and refactor generated code to match architecture |
Keep docs and tools in sync | Lint documentation, version control, and CI checks |
Wanna read more about this topic:
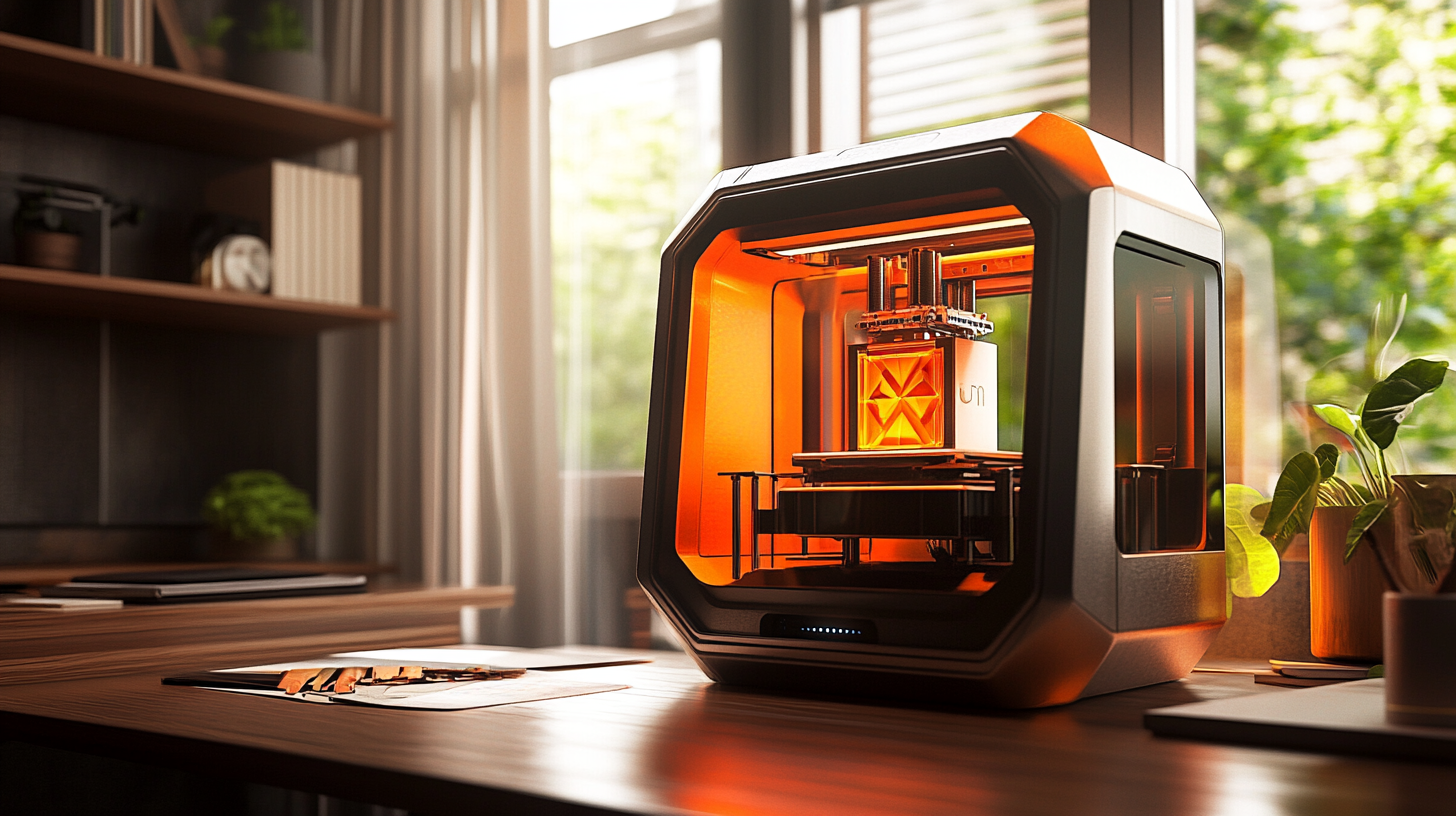
Conclusion
AI-enhanced development is no longer a novelty—it’s the new normal. Tools like GitHub Copilot, ChatGPT, Cursor, and Windsurf are shifting how code gets written, reviewed, and shipped. But with this new velocity comes a new form of technical debt: code that’s syntactically correct but structurally unsound.
The promise of Clean Architecture was never just separation for separation’s sake. It’s about creating systems that scale with clarity, testability, and resilience. In the age of AI-assisted development, those goals haven’t changed—but the strategy must evolve.
What this article has shown is that architecture must now be automated, enforced, and documented as part of your toolchain—not just your culture. Relying on human intuition or good intentions isn’t sufficient when AI can produce a hundred files in under a minute. Architectural integrity must be guarded by CI pipelines, guided by linters, and explained through documentation that’s legible to both developers and AI agents.
Let’s summarize the key takeaways:
- AI is fast, but not mindful. Without guardrails, it will introduce structural anti-patterns: leaking infrastructure into domain logic, skipping layers, and duplicating abstractions.
- Clean Architecture is your blueprint. By adopting clear, enforceable layers from day one, you create a system AI and developers alike can operate within.
- Tooling is your enforcement layer. ESLint,
eslint-plugin-boundaries
,ts-morph
, SonarQube, and GitHub Actions form the backbone of automated architectural validation. - Documentation is a signal. Structured
README.md
files, inline comments, and ADRs can guide both humans and AI toward correct architectural decisions. - Governance must evolve. Use pre-commit hooks, custom CI pipelines, bot reviewers, and labeled PRs to gate AI-generated code and enforce policy at scale.
But perhaps most important: these aren’t just defensive tactics. They’re investments in sustainable acceleration. With the right practices, AI doesn’t just speed up coding—it amplifies quality. Architecture becomes not a bottleneck, but a catalyst.
A Final Thought for AI-Native Teams
If your team is using AI to build software, you are no longer a traditional dev team. You are a hybrid team—a collaboration between humans and code-generating agents. Clean Architecture, enforced through automation and augmented with documentation, is your operating system for that collaboration.
Don’t wait to fix it later. Architect early. Automate everything. Teach your tools.
Because in the AI era, technical debt doesn’t creep—it accelerates.
Discussion