Building Universal UI Components: Embracing the Right Level of Abstraction and Zero Dependencies
Build Universal UI components with the perfect balance of abstraction, minimal dependencies, and an API-first mindset. Leverage iterative development, rigorous testing, and comprehensive documentation with Storybook to ensure reusability, maintainability, and an exceptional developer experience.
Imagine a world where every UI component you create integrates seamlessly into any project — no hacks, no hidden dependencies, no extra code.
Achieving this universality is every front-end developer’s dream. Yet, it requires a methodical approach: the right level of abstraction, an API-first mindset and minimal dependencies.
This article walks you through the principles and practices to build flexible, reusable, and maintainable UI components. Whether you’re refining a component library or setting best practices for your team, these insights will help you build robust components that developers love to use.
We’ll explore:
- Abstraction Principles
Why finding the right level is crucial. - API-First Development
Designing intuitive, minimal interfaces before diving into implementation. - Development Workflow
A step-by-step iterative process for building components. - Almost-Zero Dependencies
How reducing external dependencies maximizes compatibility. - Storybook for Documentation
Best practices for showcasing, testing, and documenting your components.
Let’s dive into the key concepts.
Understanding the Abstraction Principle
In software development, abstraction is a mental model that allows you to isolate key properties or functionalities from a bunch of implementation details. By focusing on what matters most to the context at hand, you can build solutions that are simpler to use and more adaptable in the long run.
Abstraction simplifies complexity by focusing only on the essential properties of a system. In UI component development, this principle ensures your components are simple to use, flexible, and maintainable.
What Is “The Right Level of Abstraction”?
Finding the right level of abstraction means balancing simplicity and flexibility. For example:
- Too little abstraction
A button component requiring 12 props for styling and multiple external modules makes it annoying to use & reuse. - Too much abstraction
A button component attempting to handle every edge case bloats the API and adds unnecessary complexity. - Just right
The component provides essential props (e.g.,label
,onClick
,disabled
) and a few styling variations, leaving advanced customization to the consumer.
The goal is to deliver components that focus on core functionality while empowering developers to extend or specialize them as needed.
Why Abstraction Matters
- Reusability
Well-abstracted components are easier to adapt to new projects or use cases. - Maintainability
Clear boundaries between internal logic and external interfaces reduce bugs and ease feature updates. - Consistency
Teams can rely on predictable, standardized components across projects.
API First Development
API-first development is about designing the component's public interface before implementation. This approach prioritizes the developer experience, ensuring that components are easy to adopt and maintain.
Why “API First”?
- Improved Developer Experience (DX)
Clear, intuitive APIs are easier to use and integrate. - Avoiding Feature Bloat
Designing the API upfront ensures you focus only on essential features. - Streamlined Maintenance
A well-defined, minimal API means fewer edge cases and simpler refactoring.
Applying API-First in UI Component Development
Mock the API
Write pseudo-code to define how developers will use the component. For example:
<Button label="Submit" onClick={handleSubmit} disabled={isSubmitting} />
Validate the Design
Share the API design with team members or stakeholders for feedback.
Iterate Before Implementation
Adjust the design based on feedback to address all core use cases.
Lock In
Finalize the API and begin implementation, confident that you’ve laid a solid foundation.
By focusing on the API first, you ensure a developer-friendly design that aligns with real-world needs. Keep it simple, keep it minimal.
The Development Process
Below is a recommended development flow for creating universal UI components. Notice that steps 3 (Implementation) and 4 (Testing & Validation) form an iterative loop, allowing you to refine the component repeatedly until it meets all requirements and quality standards.
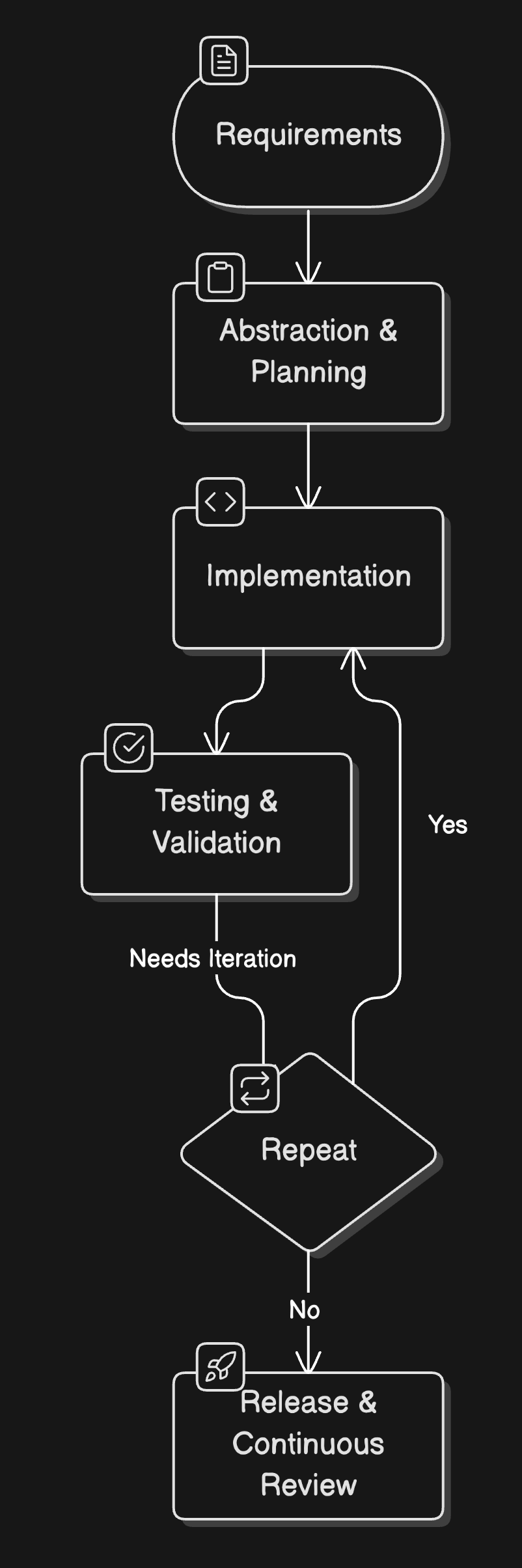
Requirements
Gather the core needs of the component:
- Purpose
What problem does it solve? - Inputs and Outputs
Based on API First design, define the minimal set of props, methods, and events. - Design Constraints
Include branding or style guidelines from your codebase.
Abstraction & Planning
Decide on the level of abstraction:
- Essential Properties
Narrow down to must-have props or methods. - Dependencies
Aim for almost zero external dependencies, using only the foundational technologies from the codebase (e.g., the codebase programming language, existing CSS framework). - Component API
Revisit the API First design to ensure all relevant use cases are covered.
Implementation (Iterative)
Begin coding the component:
- Follow Coding Standards
Maintain naming conventions and file structures. - Expose a Minimal API
Only publicize what consumers need; keep internal logic private. - Refine as Needed
You may discover nuances that require adjusting the initial plan.
Testing & Validation (Iterative)
Thoroughly test the component:
- Unit Tests
Validate each feature (render, click handlers, state changes). - Integration Tests
Ensure the component works within common parent components or frameworks. - Visual/Storybook Testing
Check how it appears and behaves under different props, states, or environments.
Note: Continue looping between Implementation and Testing & Validation until satisfied with quality and performance.
Release & Continuous Review
- Publish
Make your component available in your component library or registry. - Feedback Loop
Gather input from real-world usage and incorporate improvements. - Iterate
As new requirements emerge, revisit your component to maintain consistency and reliability.
Achieving (Almost) Zero Dependencies
When we talk about (Almost) Zero Dependencies, we mean using only the foundational technologies that your codebase already relies on — such as your chosen programming language, a standard CSS framework, and possibly few utility functions. The goal is to avoid coupling with any additional external or non-standard libraries that could limit usage or introduce complexity.
Why Aim for (Almost) Zero Dependencies?
- Wider Compatibility
With no external library ties, your component can work wherever your foundational codebase is supported. - Reduced Complexity
Fewer transitive dependencies mean fewer version conflicts and security vulnerabilities. - Lower Maintenance Cost
You won’t need to chase or patch updates for third-party libraries. - Better Cohesion with the Codebase
By aligning with your existing language and CSS framework, the component naturally fits within your project’s ecosystem.
Balancing Necessary Dependencies
- Foundational Technologies
It’s acceptable (and often required) to use your primary language (e.g., TypeScript), the project’s established CSS framework, and small utility services. - External Libraries
Avoid adopting new libraries or frameworks for just one component unless absolutely critical. When in doubt, consider a peer dependency approach, letting consumers decide which version to use.
Developing Components on Storybook
Storybook is a powerful tool for building isolated UI component catalogs. It offers a live sandbox to experiment with different states and props, making documentation and testing more interactive and visual.
Why Storybook?
- Centralized Documentation
All stories and docs live in one place, accessible to developers and designers alike. - Real-time Interaction
Quickly tweak props to see changes on the fly. - Automated Visual Testing
Tools like Chromatic or Storybook’s screenshot tests can detect UI regressions.
Which Functionalities/Behaviors Deserve a Story?
- Default State
Showcase the component in its simplest form with minimal props. - Variations & Styles
Demonstrate different visual states like primary, secondary, or danger modes. - Interactive States
Highlight events such as hover, focus, disabled, and user-triggered callbacks. - Edge Cases
Present how the component behaves with extremely long text, null data, or errors/loading states. - Combined/Composed Use Cases
If your component can be nested or contain nested elements, show real-world usage scenarios.
By covering these stories, you ensure that consumers understand how to use your component in a variety of contexts.
Best Practices for Component Documentation
- Clear Naming
Use intuitive names likeButton/Primary
orButton/Disabled
. - Readable Prop Tables
Detail each prop’s name, type, description, and default value. - Code Snippets
Include copy-paste examples for quick testing. - Contextual Tips
Provide do’s and don’ts, integration tips, and performance notes. - Versioning
Maintain a changelog for updates and breaking changes.
Conclusion
Building truly universal UI components requires a careful balance. By applying the Abstraction Principle effectively, you isolate what matters most to your component’s functionality while eliminating extraneous details. Adopting an API First Development mindset ensures you design the component’s public interface in a way that’s intuitive and minimal, driving better developer experience and maintainability.
Meanwhile, adhering to (Almost) Zero Dependencies ensures maximum portability and simplicity while leveraging only the foundational technologies of your codebase. Coupled with an iterative development flow — from requirements to abstraction, implementation/testing loops, and final release—your components will be robust, reusable, and widely compatible.
Key Takeaways:
- Right Level of Abstraction
Focus on essential inputs, outputs, and internal logic that serve your component’s purpose. - API First Development
Define the component’s consumption interface before diving into implementation to ensure a minimal, clear API. - (Almost) Zero Dependencies
Strive to minimize reliance on external libraries, using only your primary language, CSS framework, and essential utility services. - Iterative Development Flow
Requirements → Abstraction & Planning → Implementation ↔ Testing & Validation → Release & Review. - Storybook Best Practices
Document default, variation, interactive, edge, and composed states for a comprehensive reference.
When done right, these approaches result in UI components that can be effortlessly dropped into any product or project, delivering consistent and high-quality user experiences.
Discussion